add py3 soln
This commit is contained in:
parent
1153573284
commit
1e69bfd5dd
|
@ -0,0 +1,30 @@
|
||||||
|
Given the `root` of a Binary Search Tree (BST), convert it to a Greater Tree such that every key of the original BST is changed to the original key plus the sum of all keys greater than the original key in BST.
|
||||||
|
|
||||||
|
As a reminder, a _binary search tree_ is a tree that satisfies these constraints:
|
||||||
|
|
||||||
|
* The left subtree of a node contains only nodes with keys **less than** the node's key.
|
||||||
|
* The right subtree of a node contains only nodes with keys **greater than** the node's key.
|
||||||
|
* Both the left and right subtrees must also be binary search trees.
|
||||||
|
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
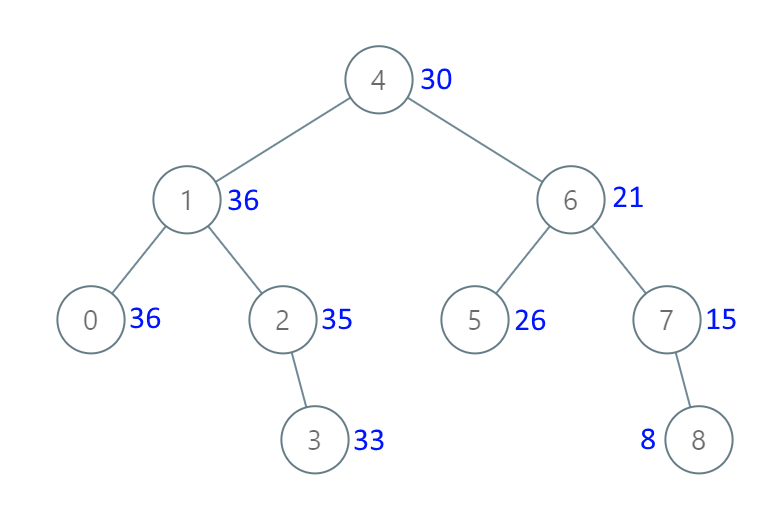
|
||||||
|
|
||||||
|
Input: root = [4,1,6,0,2,5,7,null,null,null,3,null,null,null,8]
|
||||||
|
Output: [30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
|
||||||
|
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
Input: root = [0,null,1]
|
||||||
|
Output: [1,null,1]
|
||||||
|
|
||||||
|
|
||||||
|
**Constraints:**
|
||||||
|
|
||||||
|
* The number of nodes in the tree is in the range `[0, 104]`.
|
||||||
|
* `-104 <= Node.val <= 104`
|
||||||
|
* All the values in the tree are **unique**.
|
||||||
|
* `root` is guaranteed to be a valid binary search tree.
|
||||||
|
|
||||||
|
**Note:** This question is the same as 1038: [https://leetcode.com/problems/binary-search-tree-to-greater-sum-tree/](https://leetcode.com/problems/binary-search-tree-to-greater-sum-tree/)
|
|
@ -0,0 +1,49 @@
|
||||||
|
# Definition for a binary tree node.
|
||||||
|
# class TreeNode:
|
||||||
|
# def __init__(self, val=0, left=None, right=None):
|
||||||
|
# self.val = val
|
||||||
|
# self.left = left
|
||||||
|
# self.right = right
|
||||||
|
class Solution:
|
||||||
|
# Perform reverse in-order traversal of the given BST.
|
||||||
|
# This will give us numbers in descending order as we
|
||||||
|
# touch each node.
|
||||||
|
#
|
||||||
|
# Right subtree -> Current node -> Left subtree
|
||||||
|
#
|
||||||
|
def convertBST(self, root: Optional[TreeNode]) -> Optional[TreeNode]:
|
||||||
|
self.walk(root, 0)
|
||||||
|
|
||||||
|
return root
|
||||||
|
|
||||||
|
# `total` will be the state used to keep the sum of
|
||||||
|
# all numbers greater than `node.val`
|
||||||
|
def walk(self, node, total):
|
||||||
|
if node is not None:
|
||||||
|
# Since all nums to the right will be greater
|
||||||
|
# than current, we need to get the total of
|
||||||
|
# the right subtree
|
||||||
|
right_total = self.walk(node.right, total)
|
||||||
|
|
||||||
|
# Need to store the new total based on the right
|
||||||
|
# subtree's total we obtained
|
||||||
|
next_total = node.val + right_total
|
||||||
|
|
||||||
|
# Update current node's total to its value plus
|
||||||
|
# total of all numbers greater than it (i.e right
|
||||||
|
# subtree)
|
||||||
|
node.val = next_total
|
||||||
|
|
||||||
|
# If there's a left subtree, it could mean that the
|
||||||
|
# there might be a new total that we need to return one
|
||||||
|
# level down the call stack
|
||||||
|
next_total = self.walk(node.left, next_total)
|
||||||
|
|
||||||
|
return next_total
|
||||||
|
|
||||||
|
# If node isn't present, we can't just return 0.
|
||||||
|
#
|
||||||
|
# 0 would be valid for the very first rightmost subtree.
|
||||||
|
# But as soon as we touch left subtrees, `total` could
|
||||||
|
# be different from initial 0 we pass
|
||||||
|
return total
|
Loading…
Reference in New Issue