feat(0783_search-in-a-binary-search-tree): add python3 soln
This commit is contained in:
parent
f28be89eb3
commit
27ed30d2a9
26
0783_search-in-a-binary-search-tree/README.md
Normal file
26
0783_search-in-a-binary-search-tree/README.md
Normal file
@ -0,0 +1,26 @@
|
|||||||
|
You are given the `root` of a binary search tree (BST) and an integer `val`.
|
||||||
|
|
||||||
|
Find the node in the BST that the node's value equals `val` and return the subtree rooted with that node. If such a node does not exist, return `null`.
|
||||||
|
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
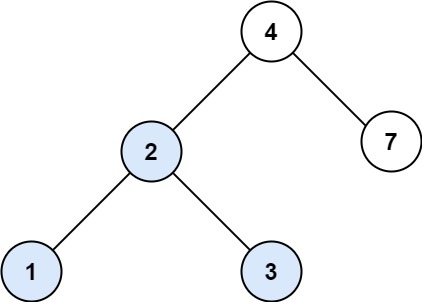
|
||||||
|
|
||||||
|
Input: root = [4,2,7,1,3], val = 2
|
||||||
|
Output: [2,1,3]
|
||||||
|
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
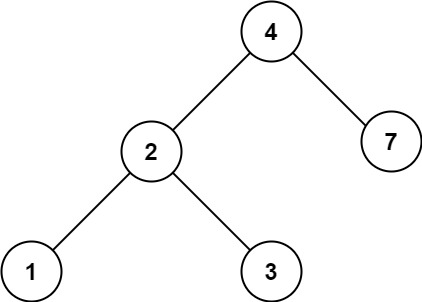
|
||||||
|
|
||||||
|
Input: root = [4,2,7,1,3], val = 5
|
||||||
|
Output: []
|
||||||
|
|
||||||
|
|
||||||
|
**Constraints:**
|
||||||
|
|
||||||
|
* The number of nodes in the tree is in the range `[1, 5000]`.
|
||||||
|
* `1 <= Node.val <= 107`
|
||||||
|
* `root` is a binary search tree.
|
||||||
|
* `1 <= val <= 107`
|
19
0783_search-in-a-binary-search-tree/python3/solution.py
Normal file
19
0783_search-in-a-binary-search-tree/python3/solution.py
Normal file
@ -0,0 +1,19 @@
|
|||||||
|
# Definition for a binary tree node.
|
||||||
|
# class TreeNode:
|
||||||
|
# def __init__(self, val=0, left=None, right=None):
|
||||||
|
# self.val = val
|
||||||
|
# self.left = left
|
||||||
|
# self.right = right
|
||||||
|
class Solution:
|
||||||
|
def searchBST(self, root: Optional[TreeNode], val: int) -> Optional[TreeNode]:
|
||||||
|
current = root
|
||||||
|
|
||||||
|
while current is not None:
|
||||||
|
if val == current.val:
|
||||||
|
return current
|
||||||
|
elif val < current.val:
|
||||||
|
current = current.left
|
||||||
|
else:
|
||||||
|
current = current.right
|
||||||
|
|
||||||
|
return None
|
Loading…
Reference in New Issue
Block a user