feat(528-swapping-nodes-in-a-ll): add py3 solution
This commit is contained in:
parent
da5d790d16
commit
2c0614e7df
23
0528_swapping-nodes-in-a-linked-list/README.md
Normal file
23
0528_swapping-nodes-in-a-linked-list/README.md
Normal file
@ -0,0 +1,23 @@
|
|||||||
|
You are given the `head` of a linked list, and an integer `k`.
|
||||||
|
|
||||||
|
Return _the head of the linked list after **swapping** the values of the_ `kth` _node from the beginning and the_ `kth` _node from the end (the list is **1-indexed**)._
|
||||||
|
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
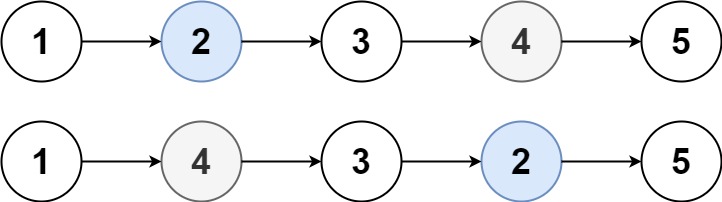
|
||||||
|
|
||||||
|
Input: head = [1,2,3,4,5], k = 2
|
||||||
|
Output: [1,4,3,2,5]
|
||||||
|
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
Input: head = [7,9,6,6,7,8,3,0,9,5], k = 5
|
||||||
|
Output: [7,9,6,6,8,7,3,0,9,5]
|
||||||
|
|
||||||
|
|
||||||
|
**Constraints:**
|
||||||
|
|
||||||
|
* The number of nodes in the list is `n`.
|
||||||
|
* `1 <= k <= n <= 105`
|
||||||
|
* `0 <= Node.val <= 100`
|
17
0528_swapping-nodes-in-a-linked-list/python3/using_dict.py
Normal file
17
0528_swapping-nodes-in-a-linked-list/python3/using_dict.py
Normal file
@ -0,0 +1,17 @@
|
|||||||
|
# NOTE: Not the best approach, since we use O(N) space
|
||||||
|
# Time: O(N)
|
||||||
|
class Solution:
|
||||||
|
def swapNodes(self, head: Optional[ListNode], k: int) -> Optional[ListNode]:
|
||||||
|
# Figure out length of LL and cache nodes by the index
|
||||||
|
length = 0
|
||||||
|
cache = {}
|
||||||
|
|
||||||
|
ptr = head
|
||||||
|
while ptr != None:
|
||||||
|
cache[length] = ptr
|
||||||
|
length += 1
|
||||||
|
ptr = ptr.next
|
||||||
|
|
||||||
|
cache[k - 1].val, cache[length - k].val = cache[length - k].val, cache[k - 1].val
|
||||||
|
|
||||||
|
return head
|
Loading…
Reference in New Issue
Block a user