search-2d-matrix py3
This commit is contained in:
parent
6925c6815f
commit
382be1e683
29
0074_search-a-2d-matrix/README.md
Normal file
29
0074_search-a-2d-matrix/README.md
Normal file
@ -0,0 +1,29 @@
|
|||||||
|
Write an efficient algorithm that searches for a value `target` in an `m x n` integer matrix `matrix`. This matrix has the following properties:
|
||||||
|
|
||||||
|
* Integers in each row are sorted from left to right.
|
||||||
|
* The first integer of each row is greater than the last integer of the previous row.
|
||||||
|
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
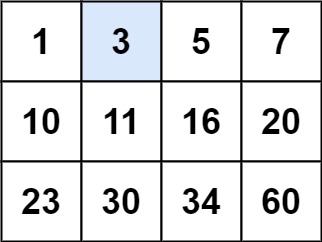
|
||||||
|
|
||||||
|
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3
|
||||||
|
Output: true
|
||||||
|
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
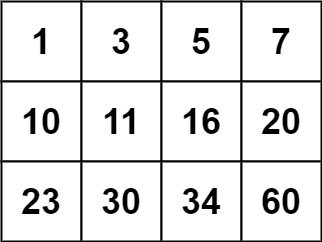
|
||||||
|
|
||||||
|
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13
|
||||||
|
Output: false
|
||||||
|
|
||||||
|
|
||||||
|
**Constraints:**
|
||||||
|
|
||||||
|
* `m == matrix.length`
|
||||||
|
* `n == matrix[i].length`
|
||||||
|
* `1 <= m, n <= 100`
|
||||||
|
* `-104 <= matrix[i][j], target <= 104`
|
||||||
|
|
||||||
|
https://leetcode.com/problems/search-a-2d-matrix/
|
42
0074_search-a-2d-matrix/python3/solution.py
Normal file
42
0074_search-a-2d-matrix/python3/solution.py
Normal file
@ -0,0 +1,42 @@
|
|||||||
|
class Solution:
|
||||||
|
def searchMatrix(self, matrix: List[List[int]], target: int) -> bool:
|
||||||
|
'''
|
||||||
|
Two binary searches, first across rows and then
|
||||||
|
across columns to quickly find the target
|
||||||
|
'''
|
||||||
|
|
||||||
|
mid = -1
|
||||||
|
start, end = 0, len(matrix) - 1
|
||||||
|
|
||||||
|
# Figure out which row the number belongs to
|
||||||
|
while start <= end:
|
||||||
|
mid = (start + end) // 2
|
||||||
|
|
||||||
|
if target > matrix[mid][-1]:
|
||||||
|
start = mid + 1
|
||||||
|
elif target < matrix[mid][0]:
|
||||||
|
end = mid - 1
|
||||||
|
else:
|
||||||
|
break
|
||||||
|
|
||||||
|
# If we never managed to find a row, that means number
|
||||||
|
# doesn't exist
|
||||||
|
if not start <= end:
|
||||||
|
return False
|
||||||
|
|
||||||
|
# The row to search
|
||||||
|
row = matrix[mid]
|
||||||
|
start, end = 0, len(row) - 1
|
||||||
|
|
||||||
|
while start <= end:
|
||||||
|
mid = (start + end) // 2
|
||||||
|
|
||||||
|
if target == row[mid]:
|
||||||
|
return True
|
||||||
|
elif target > row[mid]:
|
||||||
|
start = mid + 1
|
||||||
|
else:
|
||||||
|
end = mid - 1
|
||||||
|
|
||||||
|
return False
|
||||||
|
|
Loading…
Reference in New Issue
Block a user