same-tree py3
This commit is contained in:
parent
cccdd9269f
commit
58a19f7015
|
@ -0,0 +1,34 @@
|
|||
Given the roots of two binary trees `p` and `q`, write a function to check if they are the same or not.
|
||||
|
||||
Two binary trees are considered the same if they are structurally identical, and the nodes have the same value.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
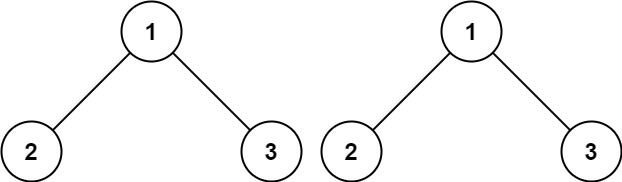
|
||||
|
||||
Input: p = [1,2,3], q = [1,2,3]
|
||||
Output: true
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
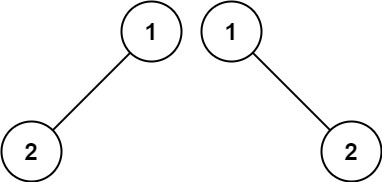
|
||||
|
||||
Input: p = [1,2], q = [1,null,2]
|
||||
Output: false
|
||||
|
||||
|
||||
**Example 3:**
|
||||
|
||||
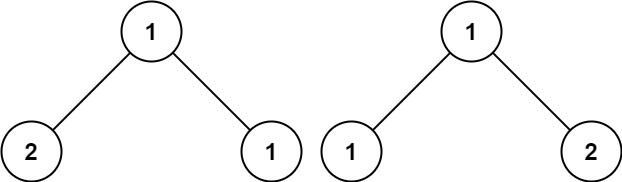
|
||||
|
||||
Input: p = [1,2,1], q = [1,1,2]
|
||||
Output: false
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* The number of nodes in both trees is in the range `[0, 100]`.
|
||||
* `-104 <= Node.val <= 104`
|
||||
|
||||
https://leetcode.com/problems/same-tree/
|
|
@ -0,0 +1,14 @@
|
|||
# Definition for a binary tree node.
|
||||
# class TreeNode:
|
||||
# def __init__(self, val=0, left=None, right=None):
|
||||
# self.val = val
|
||||
# self.left = left
|
||||
# self.right = right
|
||||
class Solution:
|
||||
def isSameTree(self, p: Optional[TreeNode], q: Optional[TreeNode]) -> bool:
|
||||
if p is None and q is None:
|
||||
return True
|
||||
elif p is None or q is None or p.val != q.val:
|
||||
return False
|
||||
|
||||
return self.isSameTree(p.left, q.left) and self.isSameTree(p.right, q.right)
|
Loading…
Reference in New Issue