spiral matrix ii
This commit is contained in:
parent
1a09f21487
commit
7129351ed4
19
0059_spiral-matrix-ii/README.md
Normal file
19
0059_spiral-matrix-ii/README.md
Normal file
@ -0,0 +1,19 @@
|
||||
Given a positive integer `n`, generate an `n x n` `matrix` filled with elements from `1` to `n2` in spiral order.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
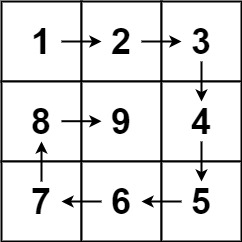
|
||||
|
||||
Input: n = 3
|
||||
Output: [[1,2,3],[8,9,4],[7,6,5]]
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
Input: n = 1
|
||||
Output: [[1]]
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* `1 <= n <= 20`
|
41
0059_spiral-matrix-ii/python3/amols_approach.py
Normal file
41
0059_spiral-matrix-ii/python3/amols_approach.py
Normal file
@ -0,0 +1,41 @@
|
||||
class Solution:
|
||||
def generateMatrix(self, n: int) -> List[List[int]]:
|
||||
if n==0: return []
|
||||
if n==1: return [[1]]
|
||||
|
||||
matrix = [[0]*n for _ in range(n)]
|
||||
counter = 1
|
||||
|
||||
rows, cols = n, n
|
||||
top, down = 0, rows-1
|
||||
left, right = 0, cols-1
|
||||
dir = 0
|
||||
|
||||
while top<=down and left<=right:
|
||||
if dir==0:
|
||||
for i in range(left, right+1):
|
||||
matrix[top][i] = counter
|
||||
counter+=1
|
||||
top+=1
|
||||
|
||||
elif dir==1:
|
||||
for i in range(top, down+1):
|
||||
matrix[i][right] = counter
|
||||
counter+=1
|
||||
right-=1
|
||||
|
||||
elif dir==2:
|
||||
for i in range(right, left-1, -1):
|
||||
matrix[down][i] = counter
|
||||
counter += 1
|
||||
down-=1
|
||||
|
||||
elif dir==3:
|
||||
for i in range(down, top-1, -1):
|
||||
matrix[i][left] = counter
|
||||
counter += 1
|
||||
left+=1
|
||||
|
||||
dir = (dir+1)%4
|
||||
|
||||
return matrix
|
40
0059_spiral-matrix-ii/python3/solution.py
Normal file
40
0059_spiral-matrix-ii/python3/solution.py
Normal file
@ -0,0 +1,40 @@
|
||||
import math
|
||||
|
||||
class Solution:
|
||||
def generateMatrix(self, n: int) -> List[List[int]]:
|
||||
m = [[None] * n for _ in range(n)]
|
||||
val = 1
|
||||
|
||||
for i in range(math.ceil(n / 2)):
|
||||
# Top
|
||||
ri, ci = i, i
|
||||
while ci < n - i:
|
||||
m[ri][ci] = val
|
||||
val += 1
|
||||
ci += 1
|
||||
ci -= 1
|
||||
|
||||
# Right
|
||||
ri, ci = ri + 1, ci
|
||||
while ri < n - i:
|
||||
m[ri][ci] = val
|
||||
val += 1
|
||||
ri += 1
|
||||
ri -= 1
|
||||
|
||||
# Bottom
|
||||
ri, ci = ri, ci - 1
|
||||
while ci >= i:
|
||||
m[ri][ci] = val
|
||||
val += 1
|
||||
ci -= 1
|
||||
ci += 1
|
||||
|
||||
# Left
|
||||
ri, ci = ri - 1, ci
|
||||
while ri > i:
|
||||
m[ri][ci] = val
|
||||
val += 1
|
||||
ri -= 1
|
||||
|
||||
return m
|
Loading…
Reference in New Issue
Block a user