maximum-depth-of-bt py3
This commit is contained in:
parent
e793d395bd
commit
713154644b
|
@ -0,0 +1,24 @@
|
||||||
|
Given the `root` of a binary tree, return _its maximum depth_.
|
||||||
|
|
||||||
|
A binary tree's **maximum depth** is the number of nodes along the longest path from the root node down to the farthest leaf node.
|
||||||
|
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
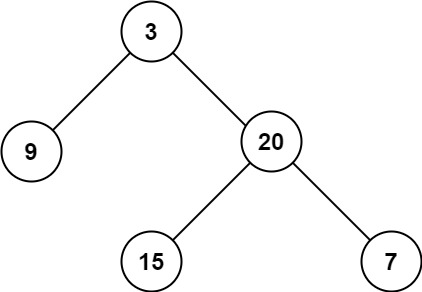
|
||||||
|
|
||||||
|
Input: root = [3,9,20,null,null,15,7]
|
||||||
|
Output: 3
|
||||||
|
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
Input: root = [1,null,2]
|
||||||
|
Output: 2
|
||||||
|
|
||||||
|
|
||||||
|
**Constraints:**
|
||||||
|
|
||||||
|
* The number of nodes in the tree is in the range `[0, 104]`.
|
||||||
|
* `-100 <= Node.val <= 100`
|
||||||
|
|
||||||
|
https://leetcode.com/problems/maximum-depth-of-binary-tree
|
|
@ -0,0 +1,27 @@
|
||||||
|
# Time: O(N)
|
||||||
|
# Space: O(N)
|
||||||
|
|
||||||
|
# Definition for a binary tree node.
|
||||||
|
# class TreeNode:
|
||||||
|
# def __init__(self, val=0, left=None, right=None):
|
||||||
|
# self.val = val
|
||||||
|
# self.left = left
|
||||||
|
# self.right = right
|
||||||
|
class Solution:
|
||||||
|
maxDepthValue = 0
|
||||||
|
|
||||||
|
def maxDepth(self, root: Optional[TreeNode]) -> int:
|
||||||
|
'''
|
||||||
|
Recursive DFS pre-order but storing max globally
|
||||||
|
'''
|
||||||
|
def findMax(node, depth):
|
||||||
|
if node is None: return None
|
||||||
|
|
||||||
|
currDepth = depth + 1
|
||||||
|
self.maxDepthValue = max(currDepth, self.maxDepthValue)
|
||||||
|
findMax(node.left, currDepth)
|
||||||
|
findMax(node.right, currDepth)
|
||||||
|
|
||||||
|
findMax(root, 0)
|
||||||
|
|
||||||
|
return self.maxDepthValue
|
|
@ -0,0 +1,17 @@
|
||||||
|
# Time: O(N)
|
||||||
|
# Space: O(N)
|
||||||
|
|
||||||
|
# Definition for a binary tree node.
|
||||||
|
# class TreeNode:
|
||||||
|
# def __init__(self, val=0, left=None, right=None):
|
||||||
|
# self.val = val
|
||||||
|
# self.left = left
|
||||||
|
# self.right = right
|
||||||
|
class Solution:
|
||||||
|
maxDepthValue = 0
|
||||||
|
|
||||||
|
def maxDepth(self, root: Optional[TreeNode]) -> int:
|
||||||
|
'''
|
||||||
|
Recursive DFS
|
||||||
|
'''
|
||||||
|
return 0 if root is None else 1 + max(self.maxDepth(root.left), self.maxDepth(root.right))
|
Loading…
Reference in New Issue