count-good-nodes in bt: add py3 soln
This commit is contained in:
parent
c53d6303b5
commit
728a3f0c82
|
@ -0,0 +1,34 @@
|
|||
Given a binary tree `root`, a node _X_ in the tree is named **good** if in the path from root to _X_ there are no nodes with a value _greater than_ X.
|
||||
|
||||
Return the number of **good** nodes in the binary tree.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
**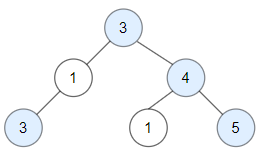**
|
||||
|
||||
Input: root = [3,1,4,3,null,1,5]
|
||||
Output: 4
|
||||
Explanation: Nodes in blue are good.
|
||||
Root Node (3) is always a good node.
|
||||
Node 4 -> (3,4) is the maximum value in the path starting from the root.
|
||||
Node 5 -> (3,4,5) is the maximum value in the path
|
||||
Node 3 -> (3,1,3) is the maximum value in the path.
|
||||
|
||||
**Example 2:**
|
||||
|
||||
**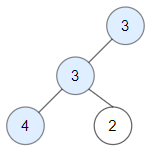**
|
||||
|
||||
Input: root = [3,3,null,4,2]
|
||||
Output: 3
|
||||
Explanation: Node 2 -> (3, 3, 2) is not good, because "3" is higher than it.
|
||||
|
||||
**Example 3:**
|
||||
|
||||
Input: root = [1]
|
||||
Output: 1
|
||||
Explanation: Root is considered as good.
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* The number of nodes in the binary tree is in the range `[1, 10^5]`.
|
||||
* Each node's value is between `[-10^4, 10^4]`.
|
|
@ -0,0 +1,37 @@
|
|||
# Time: O(N)
|
||||
# Space: O(N)
|
||||
|
||||
# Definition for a binary tree node.
|
||||
# class TreeNode:
|
||||
# def __init__(self, val=0, left=None, right=None):
|
||||
# self.val = val
|
||||
# self.left = left
|
||||
# self.right = right
|
||||
class Solution:
|
||||
def goodNodes(self, root: TreeNode) -> int:
|
||||
def dfs(node, max_value) -> int:
|
||||
'''
|
||||
Perform pre-order traversal and keep track of max
|
||||
elements in the tree. Any subsequent traversal can
|
||||
then compare against the updated max_value to see if
|
||||
it's a good node or not
|
||||
'''
|
||||
|
||||
# If no left/right nodes, then we can just return 0
|
||||
if not node: return 0
|
||||
|
||||
# Current node is good if it's value is greater than or
|
||||
# equal to the `max_value` seen so far
|
||||
res = 1 if max_value <= node.val else 0
|
||||
|
||||
# Compute the new max value, the current node could be it
|
||||
max_value = max(max_value, node.val)
|
||||
|
||||
# Do traversal on left and right nodes and add their units
|
||||
res += dfs(node.left, max_value) + dfs(node.right, max_value)
|
||||
|
||||
# This will indicate the count
|
||||
return res
|
||||
|
||||
|
||||
return dfs(root, root.val)
|
Loading…
Reference in New Issue