add py3 soln
This commit is contained in:
parent
1e69bfd5dd
commit
fdc24da4ff
26
0099_recover-binary-search-tree/README.md
Normal file
26
0099_recover-binary-search-tree/README.md
Normal file
@ -0,0 +1,26 @@
|
||||
You are given the `root` of a binary search tree (BST), where the values of **exactly** two nodes of the tree were swapped by mistake. _Recover the tree without changing its structure_.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
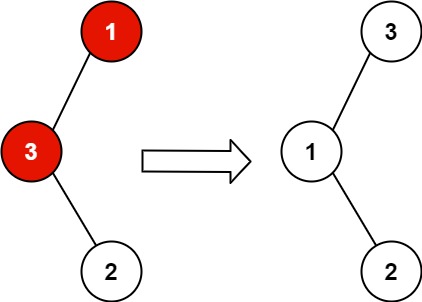
|
||||
|
||||
Input: root = [1,3,null,null,2]
|
||||
Output: [3,1,null,null,2]
|
||||
Explanation: 3 cannot be a left child of 1 because 3 > 1. Swapping 1 and 3 makes the BST valid.
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
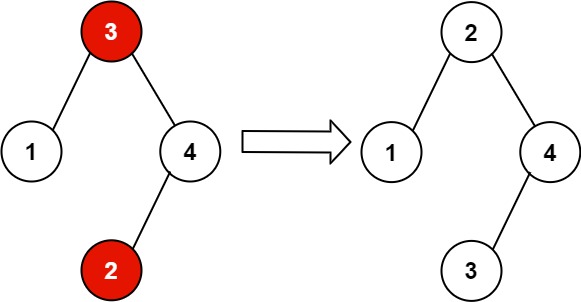
|
||||
|
||||
Input: root = [3,1,4,null,null,2]
|
||||
Output: [2,1,4,null,null,3]
|
||||
Explanation: 2 cannot be in the right subtree of 3 because 2 < 3. Swapping 2 and 3 makes the BST valid.
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* The number of nodes in the tree is in the range `[2, 1000]`.
|
||||
* `-231 <= Node.val <= 231 - 1`
|
||||
|
||||
**Follow up:** A solution using `O(n)` space is pretty straight-forward. Could you devise a constant `O(1)` space solution?
|
94
0099_recover-binary-search-tree/python3/linear_space.py
Normal file
94
0099_recover-binary-search-tree/python3/linear_space.py
Normal file
@ -0,0 +1,94 @@
|
||||
# Time: O(N)
|
||||
# Space: O(N)
|
||||
|
||||
# Definition for a binary tree node.
|
||||
# class TreeNode:
|
||||
# def __init__(self, val=0, left=None, right=None):
|
||||
# self.val = val
|
||||
# self.left = left
|
||||
# self.right = right
|
||||
class Solution:
|
||||
items = swaps = None
|
||||
|
||||
def recoverTree(self, root: Optional[TreeNode]) -> None:
|
||||
"""
|
||||
Do not return anything, modify root in-place instead.
|
||||
"""
|
||||
# Get the almost-sorted tree as an array
|
||||
self.items = self.inorder(root)
|
||||
|
||||
# Find the elements that need to be swapped
|
||||
self.swaps = self.identify_swaps(self.items)
|
||||
|
||||
# Walk the tree and fix the matching values
|
||||
self.walk_fix(root, 0)
|
||||
|
||||
|
||||
def inorder(self, node):
|
||||
"""
|
||||
Return list of elements in almost sorted order (BST property
|
||||
implies that in-order traversal produces sorted list)
|
||||
"""
|
||||
|
||||
return self.inorder(node.left) + [node.val] + self.inorder(node.right) if node is not None else []
|
||||
|
||||
|
||||
def identify_swaps(self, items):
|
||||
# We need to swap two values so keep two variables
|
||||
x = y = None
|
||||
|
||||
for i in range(len(items) - 1):
|
||||
# Problem states that we at most need to swap one item
|
||||
# at index i to another item at index j to get the sorted
|
||||
# list. If this is the case, the larger value to swap will
|
||||
# be seen first and later on, the smaller value will be seen
|
||||
|
||||
# e.g. 1 4 3 2
|
||||
# and 2 1
|
||||
if items[i] < items[i + 1]:
|
||||
pass
|
||||
else:
|
||||
# Condition fails when we see (4, 3) in first e.g and (2, 1)
|
||||
# in second example. We immediately assign i+1th value to cover
|
||||
# a case like the second example where swaps are right next to each
|
||||
# in last iteration
|
||||
y = items[i + 1]
|
||||
|
||||
# In (2, 1)'s case, immediately goes to this block and iteration stops
|
||||
# when iteration condition becomes False.
|
||||
#
|
||||
# In first example's case, we get to (4, 3) in which case:
|
||||
#
|
||||
# y = items[i + 1] = 3
|
||||
# x = items[i] = 4
|
||||
#
|
||||
# We keep looping until we hit (3, 2) and this time, it's the lower value
|
||||
# so y is reassigned to 2. x is already set previously to 4 so now we can
|
||||
# break and return the values to swap.
|
||||
if x is None:
|
||||
x = items[i]
|
||||
else:
|
||||
break
|
||||
|
||||
return {
|
||||
x: y,
|
||||
y: x
|
||||
}
|
||||
|
||||
|
||||
def walk_fix(self, node, i):
|
||||
"""
|
||||
Does in-order traversal of BST and if node.val matches
|
||||
a key in self.swaps, replaces it with the swapped value
|
||||
"""
|
||||
if node is None: return
|
||||
|
||||
self.walk_fix(node.left, i)
|
||||
|
||||
if node.val in self.swaps:
|
||||
node.val = self.swaps[node.val]
|
||||
|
||||
i += 1
|
||||
|
||||
self.walk_fix(node.right, i)
|
||||
|
0
0099_recover-binary-search-tree/python3/solution.py
Normal file
0
0099_recover-binary-search-tree/python3/solution.py
Normal file
Loading…
Reference in New Issue
Block a user