Compare commits
4 Commits
f819e84c32
...
a115f39b17
Author | SHA1 | Date | |
---|---|---|---|
a115f39b17 | |||
a0b3f7f134 | |||
0a81a963ae | |||
43b54571cf |
8
0011_container-with-most-water/NOTES.md
Normal file
8
0011_container-with-most-water/NOTES.md
Normal file
@ -0,0 +1,8 @@
|
||||
## Approaches:
|
||||
|
||||
1. Brute force $O(N^2)$
|
||||
2. Two-pointer $O(N)$
|
||||
|
||||
## Follow-up questions
|
||||
|
||||
1. What if you could slant the container?
|
22
0042_trapping-rain-water/README.md
Normal file
22
0042_trapping-rain-water/README.md
Normal file
@ -0,0 +1,22 @@
|
||||
Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
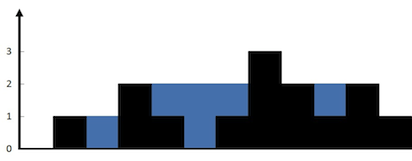
|
||||
|
||||
Input: height = [0,1,0,2,1,0,1,3,2,1,2,1]
|
||||
Output: 6
|
||||
Explanation: The above elevation map (black section) is represented by array [0,1,0,2,1,0,1,3,2,1,2,1]. In this case, 6 units of rain water (blue section) are being trapped.
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
Input: height = [4,2,0,3,2,5]
|
||||
Output: 9
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* `n == height.length`
|
||||
* `1 <= n <= 2 * 104`
|
||||
* `0 <= height[i] <= 105`
|
33
0042_trapping-rain-water/python3/imaginary_grid.py
Normal file
33
0042_trapping-rain-water/python3/imaginary_grid.py
Normal file
@ -0,0 +1,33 @@
|
||||
# Time: O(N · H) where H is the maximum height
|
||||
# Space: O(1)
|
||||
|
||||
class Solution:
|
||||
def trap(self, height: List[int]) -> int:
|
||||
maxh = 0
|
||||
|
||||
for h in height:
|
||||
maxh = max(h, maxh)
|
||||
|
||||
land_width = len(height)
|
||||
output = 0
|
||||
|
||||
for i in range(maxh):
|
||||
row_output = 0
|
||||
trough_output = 0
|
||||
trough_start = -1
|
||||
|
||||
for j in range(land_width):
|
||||
# A block is present
|
||||
if height[j] >= i + 1:
|
||||
row_output += trough_output
|
||||
trough_start = j
|
||||
trough_output = 0
|
||||
elif trough_start >= 0:
|
||||
trough_output += 1
|
||||
|
||||
output += row_output
|
||||
|
||||
return output
|
||||
|
||||
|
||||
|
0
0042_trapping-rain-water/python3/solution.py
Normal file
0
0042_trapping-rain-water/python3/solution.py
Normal file
30
0125_valid-palindrome/README.md
Normal file
30
0125_valid-palindrome/README.md
Normal file
@ -0,0 +1,30 @@
|
||||
A phrase is a **palindrome** if, after converting all uppercase letters into lowercase letters and removing all non-alphanumeric characters, it reads the same forward and backward. Alphanumeric characters include letters and numbers.
|
||||
|
||||
Given a string `s`, return `true` _if it is a **palindrome**, or_ `false` _otherwise_.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
Input: s = "A man, a plan, a canal: Panama"
|
||||
Output: true
|
||||
Explanation: "amanaplanacanalpanama" is a palindrome.
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
Input: s = "race a car"
|
||||
Output: false
|
||||
Explanation: "raceacar" is not a palindrome.
|
||||
|
||||
|
||||
**Example 3:**
|
||||
|
||||
Input: s = " "
|
||||
Output: true
|
||||
Explanation: s is an empty string "" after removing non-alphanumeric characters.
|
||||
Since an empty string reads the same forward and backward, it is a palindrome.
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* `1 <= s.length <= 2 * 105`
|
||||
* `s` consists only of printable ASCII characters.
|
23
0125_valid-palindrome/python3/solution.py
Normal file
23
0125_valid-palindrome/python3/solution.py
Normal file
@ -0,0 +1,23 @@
|
||||
class Solution:
|
||||
def isPalindrome(self, s: str) -> bool:
|
||||
i = 0
|
||||
j = len(s) - 1
|
||||
|
||||
while i < j:
|
||||
left = s[i].lower()
|
||||
right = s[j].lower()
|
||||
|
||||
if not left.isalnum():
|
||||
i += 1
|
||||
continue
|
||||
elif not right.isalnum():
|
||||
j -= 1
|
||||
continue
|
||||
|
||||
if left != right:
|
||||
return False
|
||||
|
||||
i += 1
|
||||
j -= 1
|
||||
|
||||
return True
|
36
0167_two-sum-ii-input-array-is-sorted/README.md
Normal file
36
0167_two-sum-ii-input-array-is-sorted/README.md
Normal file
@ -0,0 +1,36 @@
|
||||
Given a **1-indexed** array of integers `numbers` that is already **_sorted in non-decreasing order_**, find two numbers such that they add up to a specific `target` number. Let these two numbers be `numbers[index1]` and `numbers[index2]` where `1 <= index1 < index2 <= numbers.length`.
|
||||
|
||||
Return _the indices of the two numbers,_ `index1` _and_ `index2`_, **added by one** as an integer array_ `[index1, index2]` _of length 2._
|
||||
|
||||
The tests are generated such that there is **exactly one solution**. You **may not** use the same element twice.
|
||||
|
||||
Your solution must use only constant extra space.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
Input: numbers = [2,7,11,15], target = 9
|
||||
Output: [1,2]
|
||||
Explanation: The sum of 2 and 7 is 9. Therefore, index1 = 1, index2 = 2. We return [1, 2].
|
||||
|
||||
|
||||
**Example 2:**
|
||||
|
||||
Input: numbers = [2,3,4], target = 6
|
||||
Output: [1,3]
|
||||
Explanation: The sum of 2 and 4 is 6. Therefore index1 = 1, index2 = 3. We return [1, 3].
|
||||
|
||||
|
||||
**Example 3:**
|
||||
|
||||
Input: numbers = [-1,0], target = -1
|
||||
Output: [1,2]
|
||||
Explanation: The sum of -1 and 0 is -1. Therefore index1 = 1, index2 = 2. We return [1, 2].
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* `2 <= numbers.length <= 3 * 104`
|
||||
* `-1000 <= numbers[i] <= 1000`
|
||||
* `numbers` is sorted in **non-decreasing order**.
|
||||
* `-1000 <= target <= 1000`
|
||||
* The tests are generated such that there is **exactly one solution**.
|
15
0167_two-sum-ii-input-array-is-sorted/python3/solution.py
Normal file
15
0167_two-sum-ii-input-array-is-sorted/python3/solution.py
Normal file
@ -0,0 +1,15 @@
|
||||
class Solution:
|
||||
def twoSum(self, numbers: List[int], target: int) -> List[int]:
|
||||
left = 0
|
||||
right = len(numbers) - 1
|
||||
|
||||
while left < right:
|
||||
total = numbers[left] + numbers[right]
|
||||
|
||||
if total == target:
|
||||
return [left + 1, right + 1]
|
||||
|
||||
if total > target:
|
||||
right -= 1
|
||||
else:
|
||||
left += 1
|
33
0817_design-hashmap/README.md
Normal file
33
0817_design-hashmap/README.md
Normal file
@ -0,0 +1,33 @@
|
||||
Design a HashMap without using any built-in hash table libraries.
|
||||
|
||||
Implement the `MyHashMap` class:
|
||||
|
||||
* `MyHashMap()` initializes the object with an empty map.
|
||||
* `void put(int key, int value)` inserts a `(key, value)` pair into the HashMap. If the `key` already exists in the map, update the corresponding `value`.
|
||||
* `int get(int key)` returns the `value` to which the specified `key` is mapped, or `-1` if this map contains no mapping for the `key`.
|
||||
* `void remove(key)` removes the `key` and its corresponding `value` if the map contains the mapping for the `key`.
|
||||
|
||||
**Example 1:**
|
||||
|
||||
Input
|
||||
["MyHashMap", "put", "put", "get", "get", "put", "get", "remove", "get"]
|
||||
[[], [1, 1], [2, 2], [1], [3], [2, 1], [2], [2], [2]]
|
||||
Output
|
||||
[null, null, null, 1, -1, null, 1, null, -1]
|
||||
|
||||
Explanation
|
||||
MyHashMap myHashMap = new MyHashMap();
|
||||
myHashMap.put(1, 1); // The map is now [[1,1]]
|
||||
myHashMap.put(2, 2); // The map is now [[1,1], [2,2]]
|
||||
myHashMap.get(1); // return 1, The map is now [[1,1], [2,2]]
|
||||
myHashMap.get(3); // return -1 (i.e., not found), The map is now [[1,1], [2,2]]
|
||||
myHashMap.put(2, 1); // The map is now [[1,1], [2,1]] (i.e., update the existing value)
|
||||
myHashMap.get(2); // return 1, The map is now [[1,1], [2,1]]
|
||||
myHashMap.remove(2); // remove the mapping for 2, The map is now [[1,1]]
|
||||
myHashMap.get(2); // return -1 (i.e., not found), The map is now [[1,1]]
|
||||
|
||||
|
||||
**Constraints:**
|
||||
|
||||
* `0 <= key, value <= 106`
|
||||
* At most `104` calls will be made to `put`, `get`, and `remove`.
|
61
0817_design-hashmap/python3/solution.py
Normal file
61
0817_design-hashmap/python3/solution.py
Normal file
@ -0,0 +1,61 @@
|
||||
# Time: O(N / K) ; N is num of all keys and K is num of predefined buckets
|
||||
# Space: O(K + M) ; K is num of predefined buckets and M is no. of unique
|
||||
# keys inserted
|
||||
|
||||
from collections import deque
|
||||
|
||||
class MyHashMap:
|
||||
def __init__(self):
|
||||
# TODO: Better hash function and better initial capacity needed
|
||||
# Maybe initial capacity can even be passed as an option?
|
||||
self.store = [Bucket()] * 997
|
||||
|
||||
def put(self, key: int, value: int) -> None:
|
||||
i = self._hash(key)
|
||||
|
||||
self.store[i].upsert(key, value)
|
||||
|
||||
def get(self, key: int) -> int:
|
||||
i = self._hash(key)
|
||||
|
||||
pair = self.store[i].get_pair(key)
|
||||
|
||||
return pair[1] if pair else -1
|
||||
|
||||
def remove(self, key: int) -> None:
|
||||
i = self._hash(key)
|
||||
return self.store[i].remove(key)
|
||||
|
||||
def _hash(self, key: int):
|
||||
# TODO: Note down better hash functions
|
||||
return key % 997
|
||||
|
||||
class Bucket:
|
||||
def __init__(self):
|
||||
self.d = deque()
|
||||
|
||||
def upsert(self, key, value):
|
||||
pair = self.get_pair(key)
|
||||
|
||||
if pair is not None:
|
||||
pair[1] = value
|
||||
else:
|
||||
self.d.append([key, value])
|
||||
|
||||
def get_pair(self, key):
|
||||
for item in self.d:
|
||||
if item[0] == key:
|
||||
return item
|
||||
|
||||
def remove(self, key):
|
||||
item = self.get_pair(key)
|
||||
|
||||
if item is not None:
|
||||
self.d.remove(item)
|
||||
|
||||
|
||||
# Your MyHashMap object will be instantiated and called as such:
|
||||
# obj = MyHashMap()
|
||||
# obj.put(key,value)
|
||||
# param_2 = obj.get(key)
|
||||
# obj.remove(key)
|
Loading…
Reference in New Issue
Block a user